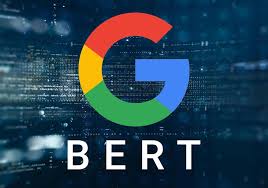
Fine-Tuning BERT for Sentiment Analysis: A Deep Learning Approach
Introduction
Sentiment analysis is the task of classifying text (such as reviews or tweets) as conveying positive or negative opinion. It’s critical in NLP: for example, movie studios use IMDb reviews to gauge audience reaction, and a well-trained classifier can analyze thousands of reviews quickly. Today, BERT (Bidirectional Encoder Representations from Transformers) is a go-to model for such tasks. Developed by Google AI in 2018, BERT is a deep bidirectional transformer that reads text in both directions, capturing rich context. By fine-tuning BERT on labeled review data, we can leverage its pre-trained language understanding to build high-accuracy sentiment classifiers.
In this article, we walk through a complete BERT sentiment analysis pipeline: data preparation, environment setup, tokenization, model building, fine-tuning, evaluation, and deployment. Along the way, you’ll learn practical tips (and even a few common pitfalls) so you can try your own BERT implementation for review classification.
Sentiment Analysis Overview
Sentiment analysis turns raw text into actionable insights by identifying opinions. Common applications include monitoring customer feedback, social media posts, or product reviews. For example, sentiment analysis lets businesses quickly determine if user feedback is positive or negative, enabling data-driven decisions.
In practice, reviews are often labeled (e.g., 0 = negative, 1 = positive) and fed to a classifier. Basic sentiment models use bag-of-words or simple embeddings, but BERT’s contextual embeddings often boost accuracy.
In short, sentiment analysis is about classifying text polarity, and using a powerful model like BERT can greatly improve results in tasks like review classification with BERT.
What is BERT?
BERT stands for Bidirectional Encoder Representations from Transformers. It’s a multi-layer Transformer encoder pre-trained on large corpora (e.g., Wikipedia, BookCorpus) using self-supervised objectives. Unlike traditional (unidirectional) language models, BERT reads text bidirectionally, meaning it learns each word’s representation from both left and right context. This deep bidirectional training allows BERT to capture nuanced meaning (like negation or sarcasm) in a sentence, which is crucial for sentiment analysis.
Importantly, BERT was trained on vast amounts of text, so it already “knows” a lot about language. We leverage this by adding a lightweight task-specific layer on top and fine-tuning on our labeled data.
In essence, BERT produces contextual embeddings (different vectors for a word depending on context), and after pre-training it only needs minimal retraining to tackle specific tasks. In this article, we focus on fine-tuning BERT for sentiment classification, but BERT can be applied to many NLP tasks with similar steps.
Preparing the Dataset
To fine-tune BERT, we need a labeled sentiment dataset. A classic example is the IMDb movie reviews: 50,000 reviews evenly split between positive and negative sentiment. (Other options include customer reviews from Amazon, Yelp, or social media posts.) Your data should have at least two columns: one for the text (review) and one for the label (e.g., 0 = negative, 1 = positive).
Before training, you should shuffle the data and optionally preprocess (e.g., lowercasing, removing HTML tags or emojis). Then split into training, validation, and test sets. A common split is 70% train, 15% validation, 15% test, using stratified sampling so both classes remain balanced in each split.
Environment Setup
We’ll use Python 3 and popular libraries. At minimum, install Hugging Face’s Transformers and a deep learning backend (PyTorch or TensorFlow), plus data tools. For example:
pip install pandas datasets scikit-learn transformers torch tensorflow
Working on a GPU (e.g., via Google Colab) is recommended since fine-tuning BERT can be slow on CPU.
Loading and Tokenizing with BERT
Next, we load the pre-trained BERT model and tokenizer:
from transformers import AutoModel, BertTokenizerFast
bert = AutoModel.from_pretrained('bert-base-uncased')
tokenizer = BertTokenizerFast.from_pretrained('bert-base-uncased')
The tokenizer splits each review into subword tokens and adds special tokens ([CLS], [SEP]) using the same vocabulary BERT was trained with. We convert all texts to the BERT input format: input IDs and attention masks, using padding and truncation.Choose a max_length (e.g., 128 tokens). Setting it too low can cut off important context. After tokenization, you’ll have tensors for input_ids, attention_mask, and labels ready for model input
Building the Model
For classification, we add a simple head on top of BERT:
from transformers import BertForSequenceClassification
model = BertForSequenceClassification.from_pretrained('bert-base-uncased', num_labels=2)
This uses the [CLS] token’s output and feeds it through a dropout layer and linear classifier.
Alternatively, build a custom PyTorch model with a dropout and dense layer. You can even freeze the BERT base if your dataset is small and only train the classification head.
Fine-Tuning BERT
We fine-tune the model using the AdamW optimizer and a small learning rate (e.g., 2e-5). BERT usually requires 2–4 epochs.
For example:
from transformers import Trainer, TrainingArguments
training_args = TrainingArguments(
output_dir='./results',
num_train_epochs=3,
per_device_train_batch_size=8,
per_device_eval_batch_size=8,
warmup_steps=500,
weight_decay=0.01,
logging_dir='./logs',
logging_steps=10,
)
trainer = Trainer(
model=model,
args=training_args,
train_dataset=train_data,
eval_dataset=val_data,
)
trainer.train()
Training on a GPU is strongly recommended for performance.
Evaluation
After training, evaluate on the test set using accuracy, precision, recall, and F1-score. A fine-tuned BERT model can achieve over 90% accuracy on balanced datasets like IMDb.
Also review the confusion matrix and classification report to understand where the model may be making errors.
Accuracy Tips
- Adjust sequence length: Avoid truncating important context. Set max_length to at least 128 tokens.
- Balance your dataset: Use class weights, oversampling, or undersampling if you have skewed labels.
- Tune hyperparameters: Adjust learning rate, batch size, and number of epochs for optimal performance.
- Regularization: Dropout (0.1–0.3) can help reduce overfitting.
- Clean data: Remove noise, duplicates, or mislabeled samples before training.
Next Steps and Webinar Invitation
Now that you’ve learned the fundamentals of fine-tuning BERT for sentiment analysis, you’re well on your way to building robust, high-performing models. Whether you’re a beginner just getting started or an expert looking to optimize further, there’s always more to explore in the world of transformer models.
Want to go hands-on and see these concepts in action? Join our upcoming free webinar, where we’ll walk you through real-time implementation of BERT for sentiment analysis and answer all your technical questions.
👉 Register now to reserve your spot.
Stay connected with us on HERE AND NOW AI & on: